Simple free shipping bar for WooCommerce + source code
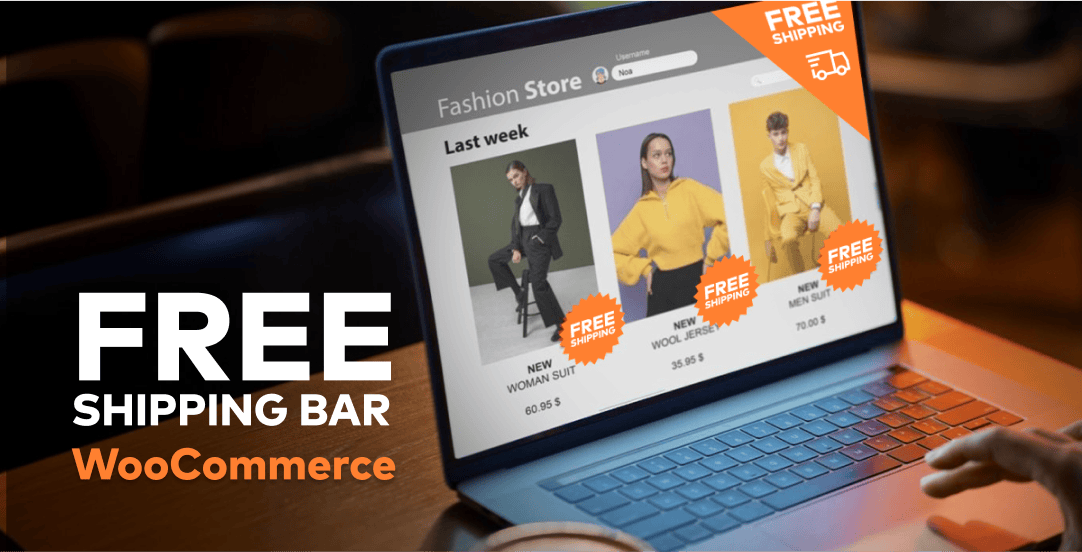
Challenge: show customers how much more they need to spend to get free shipping
Solution: a visual progress bar with live updates of the amount they need to spend to get free shipping
Have you ever wished your WooCommerce store could show customers how close they are to getting free shipping? That’s exactly what we’re going to build today – a progress bar that updates in real-time as customers add items to their cart. This feature solves a common problem in online stores: customers often don’t know how much more they need to spend to qualify for free shipping. By showing a visual progress bar, we can:
- Help customers track their progress toward free shipping
- Encourage larger purchases
- Reduce cart abandonment
- Create a better shopping experience
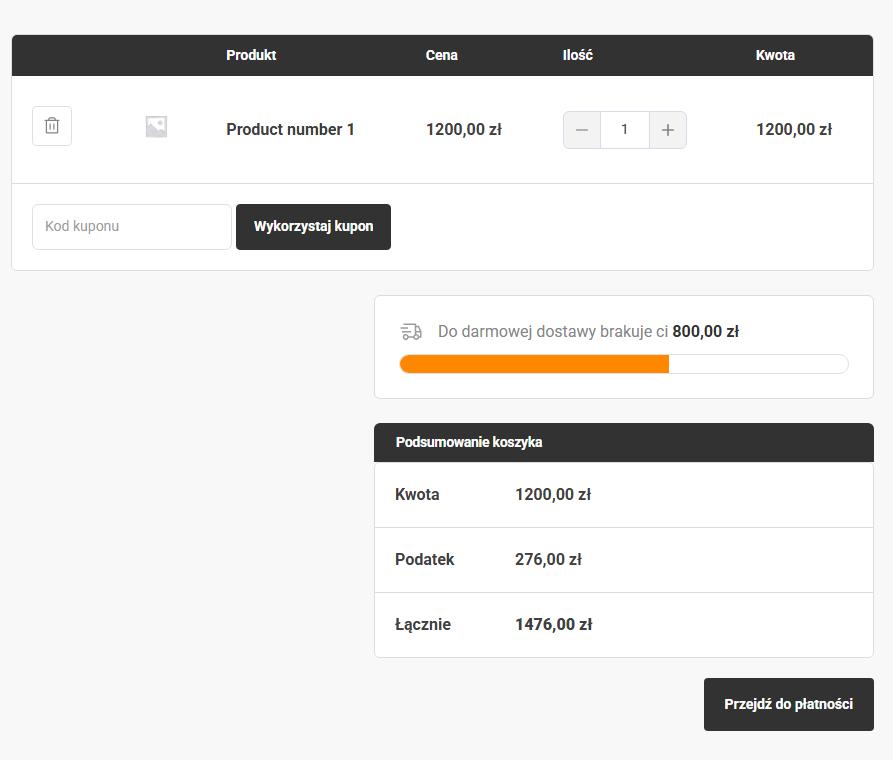
We are going to build:
- A progress bar that fills up from 0% to 100%
- Real-time updates without page refresh
- Clear messages showing the remaining amount needed
- Support for different currency and tax settings
The code we’ll look at today runs on a Polish store, but you can easily adapt it for any country or region. We’ll use standard WooCommerce hooks and WordPress functions, so you won’t need any special plugins.
Setup of free shipping progress bar in Woocommerce
Before we dive into the code, let’s check what you need to have ready:
A working WooCommerce store with:
- Shipping zones configured
- Free shipping method set up
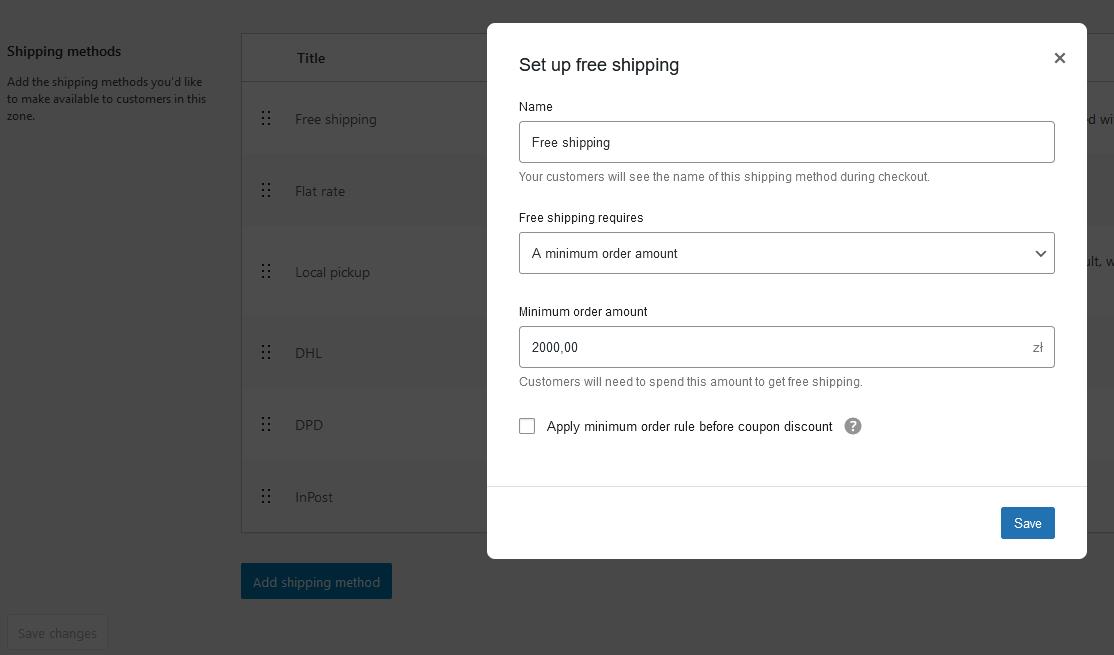
That’s it! Let’s start with the most interesting part – the code.
DIY free shipping bar with this source code
Our progress bar works in three main steps:
- It finds your store’s free shipping settings
- Calculates how much more the customer needs to spend
- Shows a nice progress bar with a message
Let’s break down each part of the code:
add_filter('woocommerce_add_to_cart_fragments', 'add_free_shipping_bar_to_fragments');
function add_free_shipping_bar_to_fragments($fragments) {
ob_start();
display_free_shipping_progress_bar();
$fragments['.free-shipping-bar-container'] = ob_get_clean();
return $fragments;
}
It makes the progress bar update whenever someone adds or removes items from their cart. It uses WooCommerce’s built-in AJAX system, so you don’t need to write any JavaScript code.
The display_free_shipping_progress_bar() function does the heavy lifting. Let’s look at its main parts:
Finding the right shipping zone:
$zones = WC_Shipping_Zones::get_zones();
$poland_zone = null;
foreach ($zones as $zone_data) {
foreach ($zone_data['zone_locations'] as $location) {
if ('PL' === $location->code && 'country' === $location->type) {
$poland_zone = $zone_data;
break 2; // Stop searching once we find Poland's shipping zone
}
}
}
// Exit if no free shipping is available
if (!$poland_zone) {
return;
}
Calculating progress:
$cart_total = WC()->cart->get_displayed_subtotal();
if ($cart_total >= $min_amount) {
$progress = 100;
$message = __('Congratulations! You get free shipping!', 'woocommerce');
} else {
$remaining = $min_amount - $cart_total;
$progress = ($cart_total / $min_amount) * 100;
$message = sprintf(
__('Add %s more for free shipping', 'woocommerce'),
'<strong>' . number_format($remaining, 2, ',', '') . ' zł</strong>'
);
}
Showing the progress bar:
echo '<div class="free-shipping-bar-container">';
echo '<div class="free-shipping-bar">';
echo '<p>' . wp_kses_post($message) . '</p>';
echo '<div class="progress-bar-container">';
echo '<div class="progress-bar" style="width: ' . esc_attr($progress) . '%;"></div>';
echo '</div>';
echo '</div>';
echo '</div>';
By default, WooCommerce displays a shipping calculator on the cart page. Since we want to focus on free shipping, it’s better to hide this calculator when customers are viewing their cart.
// Hide shipping calculator in the cart view
add_filter( 'woocommerce_cart_needs_shipping', 'filter_cart_needs_shipping' );
function filter_cart_needs_shipping( $needs_shipping ) {
if ( is_cart() ) {
$needs_shipping = false;
}
return $needs_shipping;
}
we need to display the progress bar right before the cart totals section. WooCommerce provides a hook called woocommerce_before_cart_collaterals, which allows us to insert our custom HTML before the summary section.
add_action('woocommerce_before_cart_collaterals', 'display_free_shipping_progress_bar');
function display_free_shipping_progress_bar() {
echo '<div class="free-shipping-bar-container"></div>';
}
Adding styles for better presentation (SCSS):
.free-shipping-bar {
margin: 0 0 24px 0;
padding: 24px;
background-color: #fff;
border: 1px solid #DCDCDC;
border-radius: 6px;
color:#848484;
@media (min-width: 768px) {
width: 500px;
float:right;
}
p {
margin:0 0 10px 0;
}
.progress-bar-container {
margin-top: 10px;
background-color: #fff;
border:1px solid #DFDFDF;
border-radius: 20px;
height: 20px;
overflow: hidden;
}
.progress-bar {
background-color: #FF8800;
height: 100%;
}
span.svgIcon {
float: left;
margin: 0 15px 0 0;
top: -1px;
position: relative;
}
strong {
color:#323232;
}
}
Free shipping bar without additional plugin for Woocommerce
To use this code in your store:
- Copy the code to your theme’s functions.php file
- Change ‘PL’ to your country code
- Update the messages to match your language
Now you can show your customers how much more they need to spend to get free shipping
By following these steps, we have successfully added a free shipping progress bar to the WooCommerce cart page. This simple feature can help increase average order value, improve user experience, and encourage more sales.
We used WooCommerce hooks to track cart changes and AJAX to update the display without refreshing the page. The code checks shipping zones, calculates remaining amounts, and shows a friendly message to customers. All of this happens automatically in the background.
The complete source code for this plugin is available in our GitHub repository: https://github.com/createit-dev/334-free-shipping-progress-bar-for-woocommerce